728x90
반응형
연산자 오버로딩의 이해와 유형
객체를 기본자료형처럼 연산하기 위한 수단
Point 클래스의 객체 p1, p2를 가지고, p1+p2연산은 불가능하다
하지만, operator+로 정의한다면 두가지 형태의 함수로 존재 가능하다.
멤버 함수=> 연산자의 왼쪽에 있는 피연산자를 대상으로 함수 호출
p1.operator+(p2)
전역 함수 =>
operator+(p1, p2)
연산자 오버로딩
함수 오버로딩 : fct(), fct(int)는 인자에 따라 호출되는 함수가 달라진다
연산자 오버로딩 : 3+4, p1+p2는 피연산자에 따라 연산이 달라진다.
pos1 + pos2
↓ ↓ ↓
pos1.operator+(pos2);
-> 멤버대 멤버의 덧셈 진행
멤버함수의 연산자 오버로딩
#include <iostream>
using namespace std;
class Point
{
private:
int xpos, ypos;
public:
Point(int x=0, int y=0):xpos(x),ypos(y)
{}
void ShowPostion()const
{
cout << '[' << xpos << "," << ypos <<']' << endl;
}
Point operator+(const Point& ref) //operator+라는 이름의 함수
{
Point pos(xpos + ref.xpos, ypos + ref.ypos);
return pos;
}
};
int main(void)
{
Point pos1(3, 4);
Point pos2(10, 20);
Point pos3 = pos1.operator+(pos2);
Point pos4 = pos1+pos2;
pos1.ShowPostion();
pos2.ShowPostion();
pos3.ShowPostion();
pos4.ShowPostion();
return 0;
}
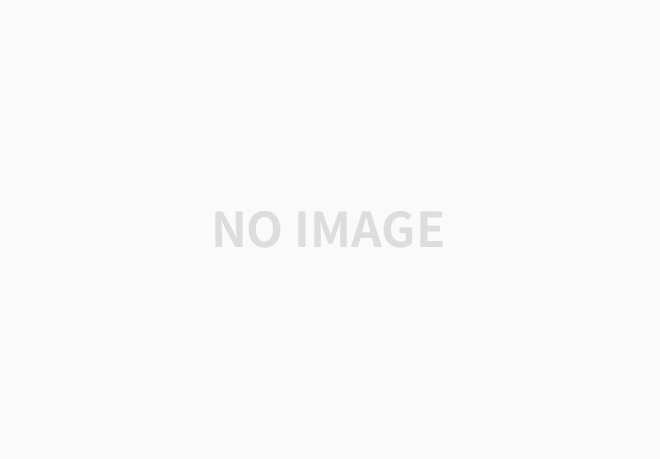
전역함수의 연산자 오버로딩
#include <iostream>
using namespace std;
class Point
{
private:
int xpos, ypos;
public:
Point(int x = 0, int y = 0) :xpos(x), ypos(y)
{}
void ShowPostion()const
{
cout << '[' << xpos << "," << ypos << ']' << endl;
}
friend Point operator+(const Point& pos1, const Point& pos2);
//함수내에서 private 영역에 접근을 허용하기 위해 friend선언
};
Point operator+(const Point& pos1, const Point &pos2)
//전역함수로 오버로딩 된 경우
{
Point pos(pos1.xpos + pos2.xpos, pos1.ypos + pos2.ypos);
return pos;
}
int main(void)
{
Point pos1(3, 4);
Point pos2(10, 20);
Point pos3 = operator+(pos1,pos2);
Point pos4 = pos1 + pos2;
pos1.ShowPostion();
pos2.ShowPostion();
pos3.ShowPostion();
pos4.ShowPostion();
return 0;
}
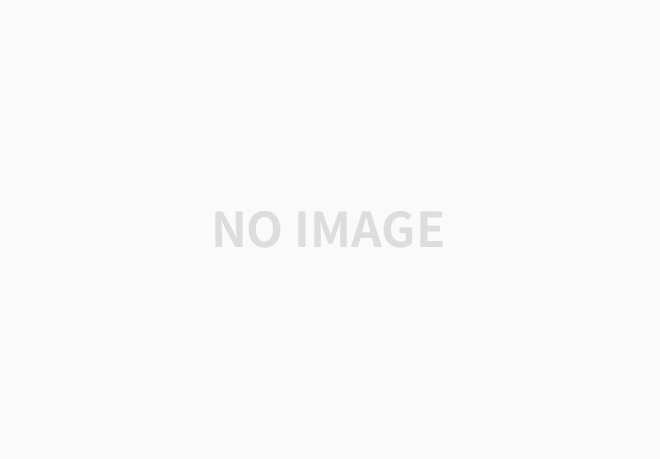
오버로딩이 불가능한 연산자의 종류
- 멤버 접근 연산자
- 멤버 포인터 연산자
- 범위 지정 연산자
- 조건 (삼항)연산자
- 바이트 단위 크기 계산 연산자
- RTTI 관련 연산자
- 형 변환 연산자
멤버함수 형태로만 오버로딩 가능한 연산자
- 대입 연산자
- 함수 호출 연산자
- 배열접근 (인덱스) 연산자
- 멤버 접근 포인터 연산자
연산자 오버로딩 주의사항
- 본래의 의도를 벗어난 형태의 오버로딩 피하기
- 연산자의 우선순위와 결합성은 바뀌지 않는다.
- 매개변수의 디폴트 값 설정 불가능
- 연산자의 순수 기능까지 빼앗을 수 없다.
728x90
반응형
'Programming > C++ 3' 카테고리의 다른 글
[C++] 15-01. 예외처리 (0) | 2021.06.09 |
---|---|
[C++] 12-01. 대입연산자 오버로딩 (0) | 2021.05.30 |
[C++] 09-02. 다중상속 (0) | 2021.05.30 |
[C++] 09-01. 멤버함수와 가상함수의 동작 원리 (0) | 2021.05.30 |
[C++] 08-03. 가상 소멸자 (0) | 2021.05.30 |