728x90
반응형
상속의 기본 조건인 IS-A 관계의 성립
- 무선 전화기 is a 전화기
- 노트북 컴퓨터 is a 컴퓨터
-> 무선 전화기는 전화기의 기본 기능에 새로운 특성이 추가
-> 노트북 컴퓨터는 컴퓨터의 기본 기능에 새로운 특성이 추가
-> is-a 관계는 논리적으로 상속을 기반으로 표현
class computer
{};
class NoteBookComp: public Computer
{};
class TabletNotebook: public NotebookComp
{};
아래로 갈수록 구체화
위로 갈수록 일반화 [범주가 넓다.]
//상속을 위한 최소한의 조건
#include <iostream>
#include <cstring>
using namespace std;
class Computer
{
private:
char owner[50];
public:
Computer(const char* name)
{
strcpy_s(owner, strlen(name)+1, name);
}
void Calculate()
{
cout << "요청 내용을 계산합니다." << endl;
}
};
class NotebookComp :public Computer
{
private:
int battery;
public:
NotebookComp(const char* name, int initChag)
:Computer(name), battery(initChag)
{
}
void Charging() { battery += 5; }
void UseBattery() { battery -= 1; }
void MovingCal()
{
if (GetBatteryInfo() < 1)
{
cout << "충전이 필요합니다." << endl;
return;
}
cout << "이동하면서 ";
Calculate();
UseBattery();
}
int GetBatteryInfo() { return battery; }
};
class TabletNotebook : public NotebookComp
{
private:
char regstPenModel[50];
public:
TabletNotebook(const char* name, int initChag,const char* pen)
:NotebookComp(name, initChag)
{
strcpy_s(regstPenModel, strlen(pen)+1, pen);
}
void Write(const char* penInfo)
{
if (GetBatteryInfo() < 1)
{
cout << "충전이 필요합니다." << endl;
return;
}
if (strcmp(regstPenModel, penInfo) != 0)
{
cout << "등록된 펜이 아닙니다.";
return;
}
cout << "필기 내용을 처리합니다." << endl;
UseBattery();
}
};
int main_1(void)
{
NotebookComp nc("이수종", 5);
TabletNotebook tn("정수영", 5, "ISE-241-242");
nc.MovingCal();
tn.Write("ISE-241-242");
return 0;
}
//이동하면서 요청 내용을 계산합니다.
//필기 내용을 처리합니다.
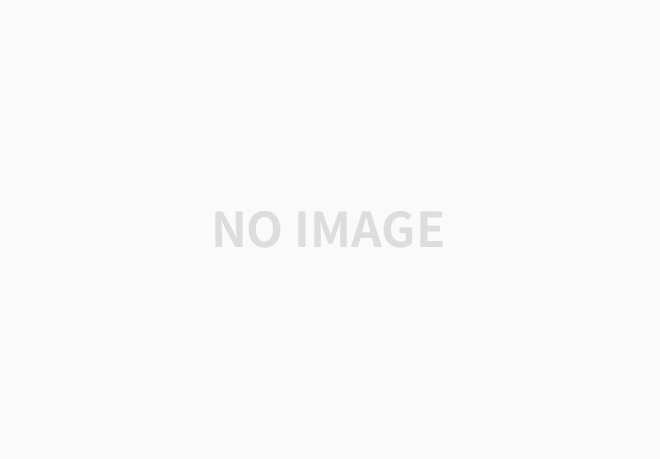
HAS-A 관계
경찰은 총을 소유한다.
경찰 had a 총
총 <- 경찰
has-a관계를 상속으로 표현하면 경찰과 총은 강한 연관성을 띠게 된다.
-> 총을 소유하지 않은 경찰이나, 다른 무기를 소유하는 경찰의 표현이 어렵다.
//Has-A 관계를 상속으로 구성하면
#include <iostream>
#include <cstring>
using namespace std;
class Gun
{
private:
int bullet;
public:
Gun(int bnum)
: bullet(bnum)
{
}
void Shut()
{
cout << "BBang!" << endl;
bullet--;
}
};
class Police :public Gun
{
private:
int handcuffs;
public:
Police(int bnum, int bcuff)
: Gun(bnum),handcuffs(bcuff)
{}
void PutHandCuff()
{
cout << "SNAP!" << endl;
handcuffs--;
}
};
int main(void)
{
Police pman(5, 3);
pman.Shut();
pman.PutHandCuff();
return 0;
}
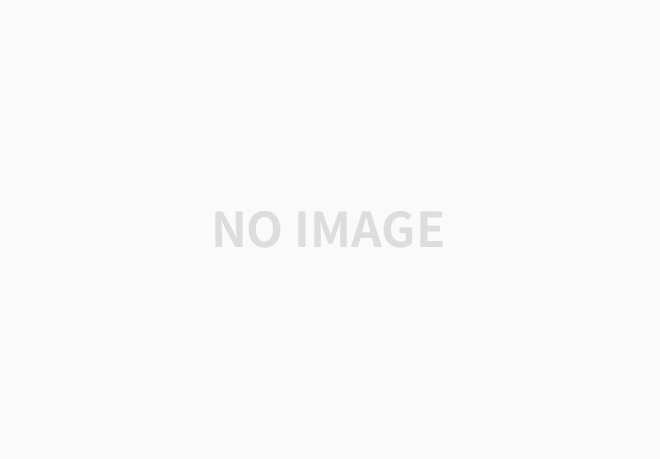
//HAS-A 관계를 포함으로 표현
#include<iostream>
#include<cstring>
using namespace std;
class Gun
{
private:
int bullet; //장전된 총알의 수
public:
Gun(int bnum):bullet(bnum)
{}
void Shut()
{
cout << "BBANG!" << endl;
bullet--;
}
};
class Police
{
private:
int handcuffs; //소유한 수갑의 수
Gun* pistol; //소유하고 있는 권총 -> 포인터형으로 포함관게 표현
//총이라는 클래스를 멤버로 포함 -> 연관성은 낮고, 변경이 용이
public:
Police(int bnum, int bcuff) //생성자의 조건에 따라 총 포함여부 결정
:handcuffs(bcuff)
{
if (bnum > 0)
pistol = new Gun(bnum);
else
pistol = NULL;
}
void PutHandCuff()
{
cout << "SNAP!" << endl;
handcuffs--;
}
void Shut()
{
if (pistol == NULL)
cout << "Hut BBANG!" << endl;
else
pistol->Shut();
}
~Police()
{
if (pistol != NULL)
delete pistol;
}
};
int main_3(void)
{
Police pman1(5, 3);
pman1.Shut();
pman1.PutHandCuff();
Police pman2(0, 3);
pman2.Shut();
pman2.PutHandCuff();
return 0;
}
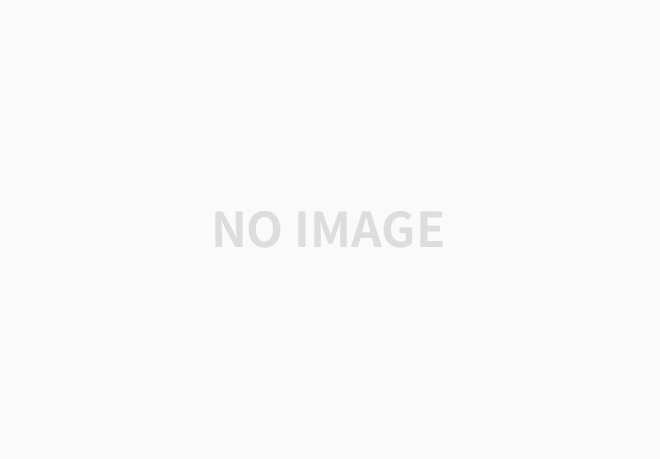
728x90
반응형
'Programming > C++ 3' 카테고리의 다른 글
[C++] 08-02. 가상함수 [급여관리 시스템 3단계] (0) | 2021.05.29 |
---|---|
[C++] 08-01. 객체 포인터의 참조관계 [급여관리 시스템 2단계] (0) | 2021.05.29 |
[C++] 07-03. protected 선언과 세 가지 형태의 상속 (0) | 2021.05.29 |
[C++] 07-02. 상속의 문법적인 이해 (0) | 2021.05.29 |
[C++] 07-01. 상속에 대한 이해와 접근 [급여관리시스템 1단계] (0) | 2021.05.28 |