728x90
반응형
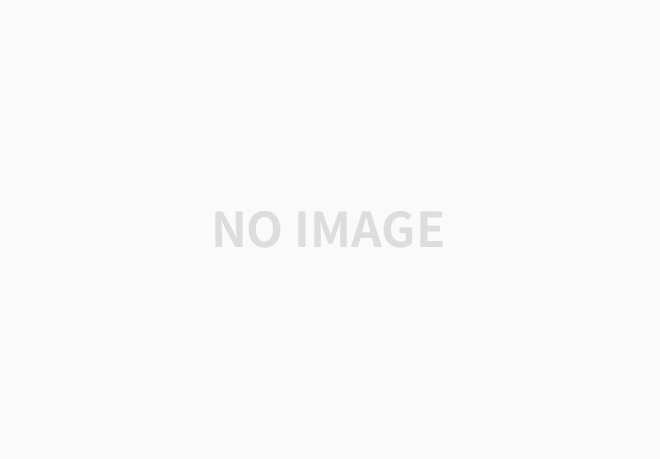
Object 클래스
-
- 참조값만 비교
- 객체 자신과 파라미터로 전달된 객체를 비교 참조 값을 비교
- equals() 메서드를 오버라이딩해서 객체의 값을 비교하는 것으로 변경한다.
package ch13.objequals;
public class ObjectEqualsTest {
public static void main(String args[]) {
Object obj1 = new Object();
Object obj2 = new Object();
if(obj1.equals(obj2)) { // obj1 == obj2
System.out.println("obj1.equals(obj2) -> true");
} else {
System.out.println("obj1.equals(obj2) -> false");
}
ObjectEq oeq1 = new ObjectEq(10);
ObjectEq oeq2 = new ObjectEq(10);
// 객체 참조값을 비교
if(oeq1 == oeq2) {
System.out.println("o1 == o2 -> true");
} else {
System.out.println("o1 == o2 -> false");
}
// 오바라이딩된 메서드를 호출하여 객체의 필드값을 비교
if(oeq1.equals(oeq2)) {
System.out.println("o1.equals(o2) -> true");
}
}
}
class EqualsEx1 {
public static void main(String[] args) {
Value v1 = new Value(10);
Value v2 = new Value(10);
if (v1.equals(v2)) {
System.out.println("v1과 v2는 같습니다.");
} else {
System.out.println("v1과 v2는 다릅니다.");
}
v2 = v1;
if (v1.equals(v2)) {
System.out.println("v1과 v2는 같습니다.");
} else {
System.out.println("v1과 v2는 다릅니다.");
}
} // main
}
class Value {
int value;
Value(int value) {
this.value = value;
}
}
package ch13.objequals;
class ObjectEq {
private int val;
public ObjectEq(int val){
this.val = val;
}
@Override
public boolean equals(Object obj) {
if(obj != null && obj instanceof ObjectEq) {
return this.val == ((ObjectEq)obj).val;
}
return false;
}
}
class Person {
long id;
public boolean equals(Object obj) {
if(obj!=null && obj instanceof Person) {
return id ==((Person)obj).id;
} else {
return false;
}
}
Person(long id) {
this.id = id;
}
}
class EqualsEx2 {
public static void main(String[] args) {
Person p1 = new Person(8011081111222L);
Person p2 = new Person(8011081111222L);
if(p1==p2)
System.out.println("p1과 p2는 같은 사람입니다.");
else
System.out.println("p1과 p2는 다른 사람입니다.");
if(p1.equals(p2))
System.out.println("p1과 p2는 같은 사람입니다.");
else
System.out.println("p1과 p2는 다른 사람입니다.");
}
}
- 얕은 복사와 깊은 복사
import java.util.*;
class Circle implements Cloneable {
Point2 p; // 원점
double r; // 반지름
Circle(Point2 p, double r) {
this.p = p;
this.r = r;
}
public Circle shallowCopy() { // 얕은 복사
Object obj = null;
try {
obj = super.clone();
} catch (CloneNotSupportedException e) {}
return (Circle)obj;
}
public Circle deepCopy() { // 깊은 복사
Object obj = null;
try {
obj = super.clone();
} catch (CloneNotSupportedException e) {}
Circle c = (Circle)obj;
c.p = new Point2(this.p.x, this.p.y);
return c;
}
public String toString() {
return "[p=" + p + ", r="+ r +"]";
}
}
class Point2 {
int x;
int y;
Point2(int x, int y) {
this.x = x;
this.y = y;
}
public String toString() {
return "("+x +", "+y+")";
}
}
class ShallowCopy {
public static void main(String[] args) {
Circle c1 = new Circle(new Point2(1, 1), 2.0);
Circle c2 = c1.shallowCopy();
Circle c3 = c1.deepCopy();
System.out.println("c1="+c1);
System.out.println("c2="+c2);
System.out.println("c3="+c3);
c1.p.x = 9;
c1.p.y = 9;
System.out.println("= c1의 변경 후 =");
System.out.println("c1="+c1);
System.out.println("c2="+c2);
System.out.println("c3="+c3);
}
}
- hashCode()
- 객체 자신의 해시코드를 반환
- 컬렉션 객체인 HashSet에서는 equals()를 오버라이딩할때 함께 오버라이딩해줘야한다.
import java.util.*;
class HashSetEx4 {
public static void main(String[] args) {
HashSet set = new HashSet();
set.add(new String("abc"));
set.add(new String("abc"));
set.add(new Person2("David",10));
set.add(new Person2("David",10));
System.out.println(set);
}
}
class Person2 {
String name;
int age;
Person2(String name, int age) {
this.name = name;
this.age = age;
}
public boolean equals(Object obj) {
if(obj instanceof Person2) {
Person2 tmp = (Person2)obj;
return name.equals(tmp.name) && age==tmp.age;
}
return false;
}
public int hashCode() {
return (name+age).hashCode();
}
public String toString() {
return name +":"+ age;
}
}
- getClass()
- 현재 자신의 객체 클래스 정보를 가지고 있는 런타임 클래스 객체를 얻을 수 있다.
- 리플렉션 API를 이용하면 클래스 텍스트명으로 클래스 객체 생성, 메서드 호출도 가능하다.
Class 클래스의 인스턴스를 런타임 클래스 객체라고 한다.
-> 리소스가 많이 소요되므로 비즈니스로직에서는 사용을 자제해야한다.
- Class<?> clazz = Class.forName("클래스경로")를 이용하면 접근제어자를 무시하고 클래스 정보를 가져올 수 있다.
- getName()
- getFields()
- getConstructor()
- getMethods()
package ch13.objgetclass;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
public class GetClassEx1 {
public static void main(String args[]) {
String str = "getClass() 메서드 실행";
Class clazz = str.getClass();
System.out.println("클래스명 : " + clazz.getName());
System.out.println("상위 클래스명 : " + clazz.getSuperclass());
System.out.println("메서드 목록");
for(Method method : clazz.getMethods()){
System.out.print(method.getName() + "\t");
}
System.out.println();
System.out.println("필드 목록");
for(Field field : clazz.getFields()){
System.out.print(field.getName() + "\t");
}
}
}
System Properties (환경 변수)
- getProperty()
- setProperty()
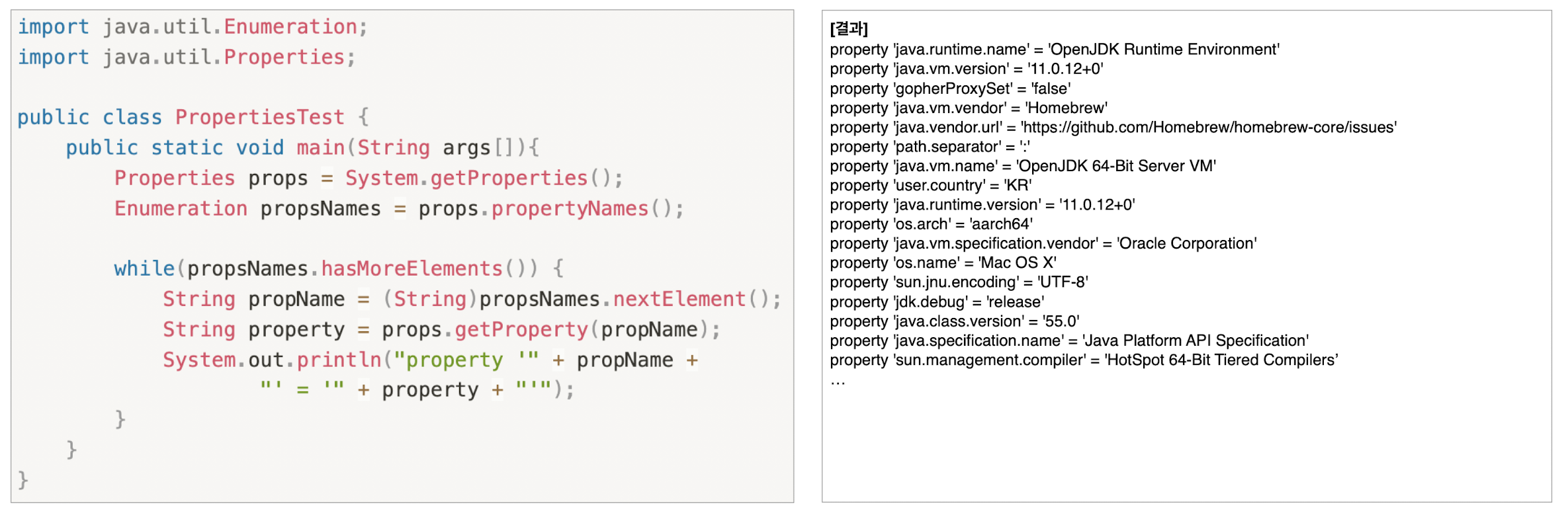
package ch13.prop;
import java.io.FileWriter;
import java.util.Properties;
public class PropsTestEx2 {
public static void main(String[] args)throws Exception{
Properties p=new Properties();
p.setProperty("name","홍길동");
p.setProperty("email","perfectjava@naver.com");
p.store(new FileWriter("info.properties"),"Properties Example");
}
}
package ch13.prop;
import java.util.Enumeration;
import java.util.Properties;
public class PropertiesTest {
public static void main(String args[]){
Properties props = System.getProperties();
Enumeration propsNames = props.propertyNames();
while(propsNames.hasMoreElements()) {
String propName = (String)propsNames.nextElement();
String property = props.getProperty(propName);
System.out.println("property '" + propName +
"' = '" + property + "'");
}
}
}
Math class
- 모두 static 메서드로 객체 생성없이 사용
- 상수로 PI, E가 존재
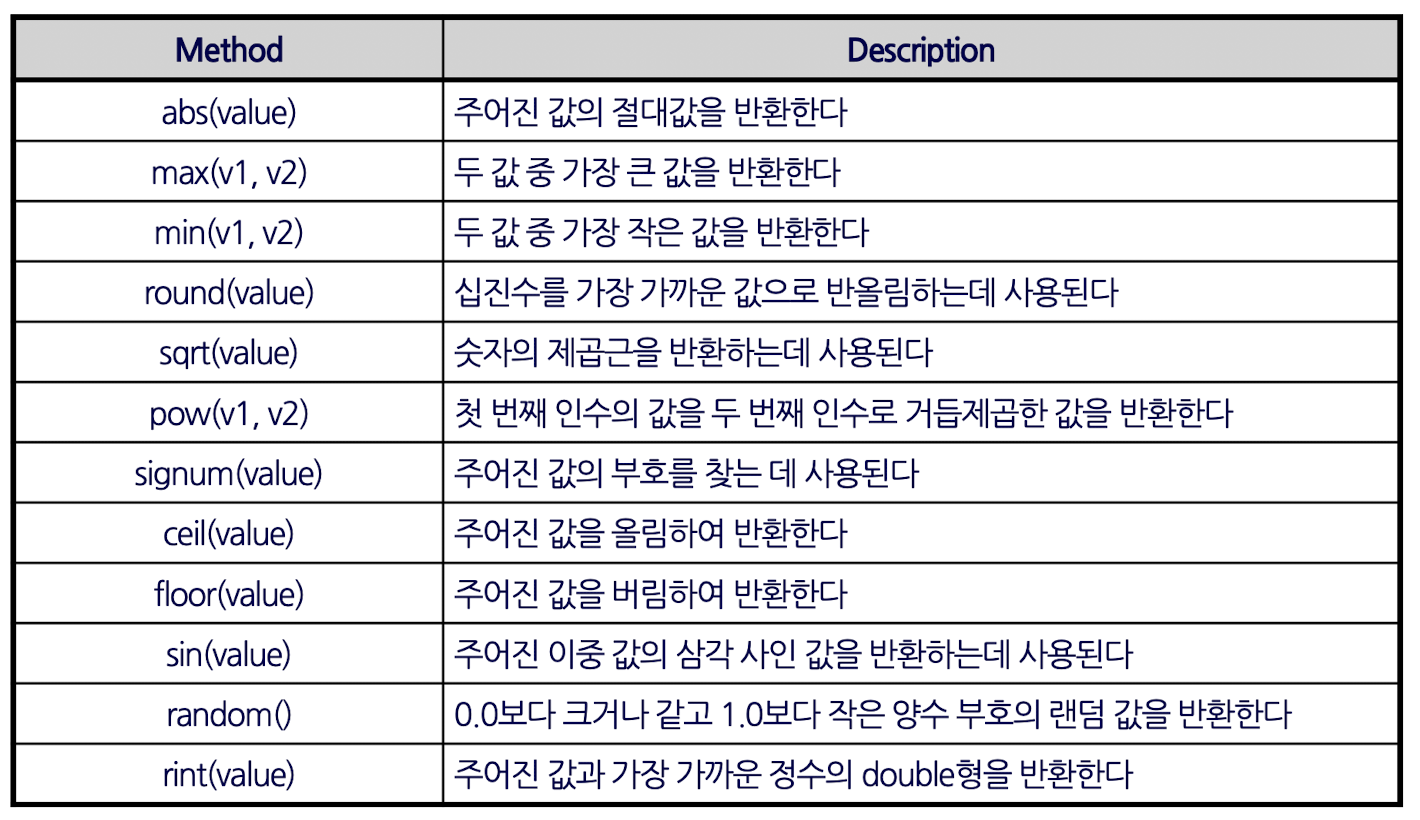
package ch13;
import static java.lang.System.*;
public class MathTest {
public static void main(String args[]){
out.println("10의 절대값 : " + Math.abs(10));
out.println("10.0 절대값 : " + Math.abs(10L));
out.println("20.0d의 절대값 : " + Math.abs(-20.0d));
out.println("큰 값을 반환 : " + Math.max(20, 10));
out.println("작은 값을 반환 : " + Math.min(10.0, 20.0));
out.println("Random 값을 반환 : " + Math.random());
out.println("올림 값 : " + Math.ceil(20.3));
out.println("작거나 같은 가장 작은 정수를 반환 : " + Math.floor(99.7));
out.println("가장 가까운 정수를 double 타입으로 반환 : " + Math.rint(101.57));
out.println("radians로 지정된 sin 값을 반환 : " + Math.sin(100));
out.println("radians로 지정된 cos 값을 반환 : " + Math.cos(100));
out.println("radians로 지정된 tan 값을 반환 : " + Math.tan(100));
out.println("100으로 지정된 로그값을 반환 : " + Math.log(100));
out.println("3.0의 2.0승 값을 double 값으로 반환 : " + Math.pow(3.0, 2.0));
out.println("Square root값을 double 값으로 반환 : " + Math.sqrt(3));
}
}
Wrapper class
- 기본형 값을 객체로 다룰 때 사용
- Java5부터 오토박싱과 언박싱으로 자동변환을 지원한다.
- 언박싱 시에는 static메서드인 valueOf()
- instanceof 연산자를 이용해 확인 후 형변환해서 사용한다.
- Wrapper 클래스의 조상클래스인 Number클래스의 메서드 사용
- intValue()
- doubleValue()
- longValue()
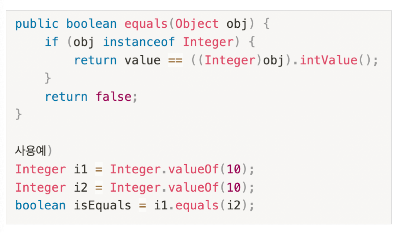
package ch13.wrapper;
public class Boxing {
public static void main(String arggs[]){
int val = 20;
// valueOf() 메서드를 이용하여 래퍼 클래스 객체 변환
Integer i1 = Integer.valueOf(val);
// Autoboxing으로 래퍼 클래스 객체 자동 변환
Integer i2 = val;
}
}
package ch13.wrapper;
public class UnBoxing {
public static void main(String arggs[]){
// new 생성자를 사용하는 것은 deprecated 되었다
// Integer i = new Integer(20);
Integer i = Integer.valueOf(20); // 박싱
// intValue() 메서드를 이용하여 기본형으로 변환
int val1 = i.intValue();
// AutoUnboxing 기능으로 기본형으로 자동 변환
int val2 = i;
}
}
package ch13.wrapper;
public class AutoBoxingUnBoxing {
public static void main(String arggs[]) {
Integer num = Integer.valueOf(15); // 박싱
int n = num.intValue(); // 언박싱
System.out.println(n);
Character ch = 'X'; // Character ch = new Character('X'); : 오토박싱
char c = ch; // char c = ch.charValue(); : 오토언박싱
System.out.println(c);
// 오토박싱과 오토언박싱을 통해 기본형과 래퍼클래스간의 다양한 연산도 가능하다
Integer num1 = Integer.valueOf(7); // 박싱
Integer num2 = Integer.valueOf(3); // 박싱
int int1 = num1.intValue(); // 언박싱
int int2 = num2.intValue(); // 언박싱
Integer result1 = num1 + num2; // 10
Integer result2 = int1 - int2; // 4
}
}
package ch13.wrapper;
public class WrapperClassEx01 {
public static void main(String args[]){
Integer i1 = Integer.valueOf(10);
Integer i2 = Integer.valueOf(10);
boolean isEquals1 = i1.equals(i2);
boolean isEquals2 = i1 == i2;
System.out.println(isEquals1);
System.out.println(isEquals2);
}
}
package ch13.wrapper;
public class WrapperClassEx02 {
public static void main(String args[]){
byte b=20;
short s=10;
int i=70;
long l=200;
float f=10.0F;
double d=20.0D;
char c='c';
boolean bool=true;
// Autoboxing
Byte byteobj=b;
Short shortobj=s;
Integer intobj=i;
Long longobj=l;
Float floatobj=f;
Double doubleobj=d;
Character charobj=c;
Boolean boolobj=bool;
System.out.println("랩퍼 클래스 객체");
System.out.println("Byte object :"+ byteobj);
System.out.println("Short object: "+ shortobj);
System.out.println("Integer object: "+ intobj);
System.out.println("Long object: "+ longobj);
System.out.println("Float object: "+ floatobj);
System.out.println("Double object: "+ doubleobj);
System.out.println("Character object: "+ charobj);
System.out.println("Boolean object: "+ boolobj);
// Unboxing
byte bytevalue = byteobj;
short shortvalue = shortobj;
int intvalue = intobj;
long longvalue = longobj;
float floatvalue = floatobj;
double doublevalue = doubleobj;
char charvalue = charobj;
boolean boolvalue = boolobj;
System.out.println("기본형(Primitive type)");
System.out.println("byte value: " + bytevalue);
System.out.println("short value: " + shortvalue);
System.out.println("int value: " + intvalue);
System.out.println("long value: " + longvalue);
System.out.println("float value: " + floatvalue);
System.out.println("double value: " + doublevalue);
System.out.println("char value: " + charvalue);
System.out.println("boolean value: " + boolvalue);
}
}
Number클래스
- 4개의 추상 메서드와 2개의 메서드 제공
- 객체를 다른 기본형으로의 변환도 가능하다 (실수 -> 정수)
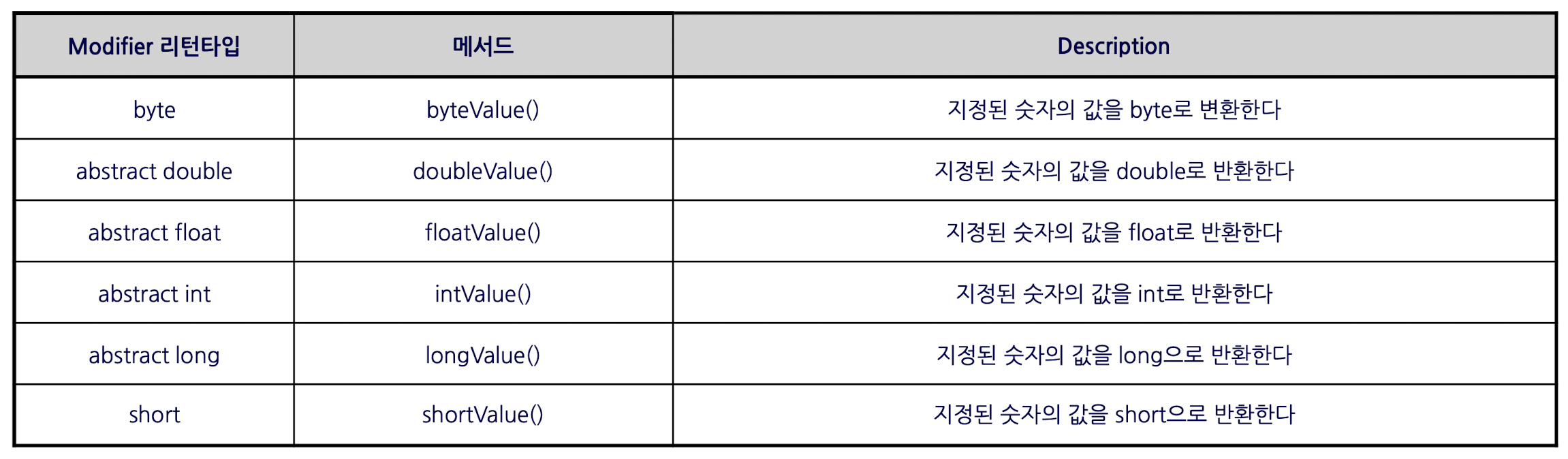
package ch13.wrapper;
public class NumberTestEx {
public static void main(String args[]){
Double doubleValue = Double.valueOf(123.456);
int intValue = doubleValue.intValue(); // 소숫점 버림
short shortValue = doubleValue.shortValue();
long longValue = doubleValue.longValue();
System.out.println("integer value: " + intValue);
System.out.println("short value: " + shortValue);
System.out.println("long value: " + longValue);
}
}
String class
- char 값의 시퀀스를 나타내느 객체로 내부적으로 ch[]로 구성된다.
- compare(), concat(), equals(), split(), length(), replace(), compareTo(), intern(), substring() 문자열 작업 수행하는 메서드가 존재한다.
- Serializable, Comparable, CharSequence 인터페이스를 구현한다.
- String 객체의 인스턴스 내용은 변경 불가능하므로, immutable object라고 하며, 문자열이 변경될 때마다 새로운 인스턴스가 생성된다.
- CharSequence 인터페이스는 문자 시퀀스를 나타내는데 사용되며, char 값을 읽을 수 있는 시퀀스
- 변경 가능한 문자열은 StringBuffer, StringBuilder 클래스를 사용할 수 있다.
- 쓰레드로부터 보호 = StringBuffer [Thread-Safety]
- 쓰레드로부터 안전하지 않다 = StringBuilder
- 문자열은 큰 따옴표를 사용해 생성하는데, 문자열 객체는 string constant pool이라는 특별한 메모리 영역에 저장
- 문자열 리터럴 생성시마다 JVM이 풀을 확인해 동일한 문자열이 존재하면 참조값을 반환하고, 없으면 인스턴스를 생성해 풀에 배치한다.
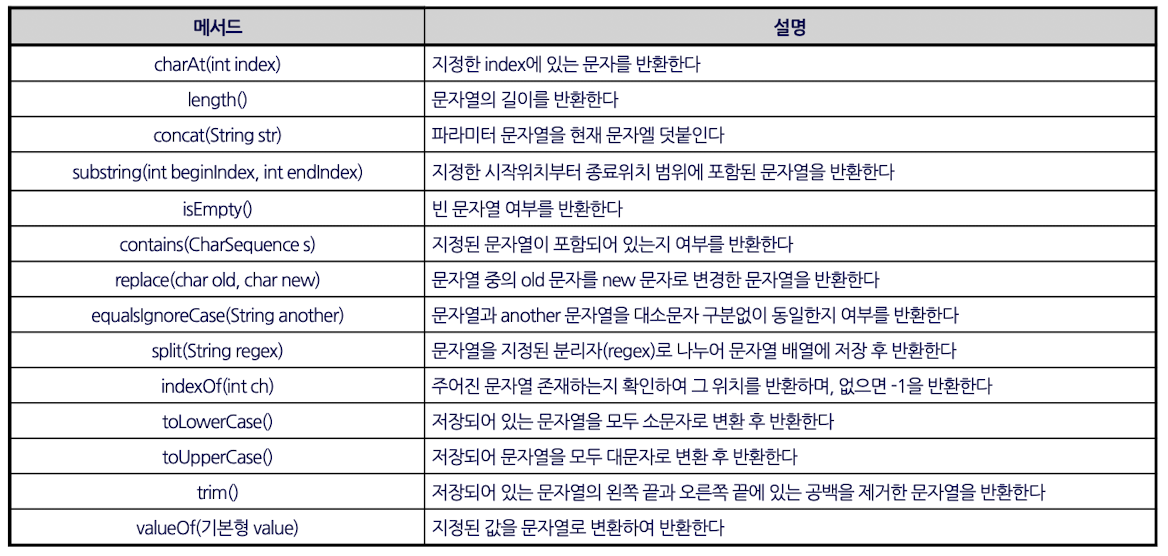
package ch13.string;
public class StringEx01 {
public static void main(String args[]){
String s1 = "java"; // 문자열 리터럴로 string 객체 생성
char ch[] = {'g','i','l','d','o','n','g'};
String s2 = new String(ch); // char 배열을 문자열로 변환
// new 키워드로 자바 문자열 객체 생성
String s3 = new String("새로운 문자열");
System.out.println(s1);
System.out.println(s2);
System.out.println(s3);
String strVar1 = "abc";
String strVar2 = "abc";
String strVar3 = new String("abc");
String strVar4 = new String("abc");
// true = 동일한 객체 참조값
System.out.println(strVar1 == strVar2);
// false = new로 생성되어 객체 참조값이 다르다
System.out.println(strVar3 == strVar4);
// 오버라이된 equals메서드로 값을 비교
System.out.println(strVar1.equals(strVar2));
System.out.println(strVar3.equals(strVar4));
}
}
package ch13.string;
import java.util.Locale;
public class StringEx02 {
public static void main(String args[]){
String s = new String("ABCDEFGH");
System.out.println(s.charAt(4)); // E
System.out.println(s.compareTo("ABCDEFGH")); // 0
System.out.println(s.compareToIgnoreCase("abcdefgh")); // 0
System.out.println(s.concat("abc")); // ABCDEFGHabc
System.out.println(s.endsWith("FGH")); // true
System.out.println(s.equals("ABCDEFGH")); // true
System.out.println(s.equalsIgnoreCase("abcdefgh")); // true
s = new String("This is a String");
System.out.println(s.indexOf("i")); // 2
System.out.println(s.indexOf("i", 7)); // 13
System.out.println(s.indexOf("is")); // 2
System.out.println(s.lastIndexOf("is")); // 5
System.out.println(s.length()); // 16
System.out.println(s.replace('i', 'Q')); // ThQs Qs a StrQng
System.out.println(s.replaceAll("is", "IS")); // thIS IS a String
System.out.println(s.startsWith("This")); // true
System.out.println(s.substring(5)); // is a String
System.out.println(s.substring(5, 13)); // is a Str
System.out.println(s.toLowerCase()); // this is a string
System.out.println(s.toUpperCase()); // THIS IS A STRING
}
}
#trim()은 모든 공백을 지우지만 java11부터 앞의 공백만, 뒤의 공백만 지우는 메서드 추가
- stripLeading()
- 앞의 공백만 지운다.
- stripTrailing()
- 뒤의 공백만 지운다.
replaceAll에 정규식으로 뒤의 공백 제거하는 방법
String str = " ABCD ";
str = str.replaceAll("\\s+$", "");
StringBuffer 클래스
- 동일한 객체에 값을 변경가능한 변경가능한 객체
- 멀티스레드 환경에서 불변 객체인 String의 경우에는 값이 변하지 않는다는 점이 보장되므로 스레드 세이프 처리를 하지 않아도 된다.변경가능한 객체인 StringBuffer의 경우에 스레드 세이프한 처리한 코드를 가지고 있다. (즉, syncronized- 동기화 처리 수행)
변경가능한 객체인 StringBuilder의 경우에 스레드 세이프한 처리가 되어있지 않다. - 생성자
- StringBuffer() : 빈 버퍼
- StringBuffer(int capacity) : 매개변수만큼 버퍼를 초기화해 생성
- StringBuffer(String initialString) : 초기 스트링 값을 포함해 버퍼를 초기화해 생성
- 수정 메서드
- append
- insert
- reverse
- setCharAt
- setLength
- append()메서드에 스레드 동기화를 위한 synchronized 처리
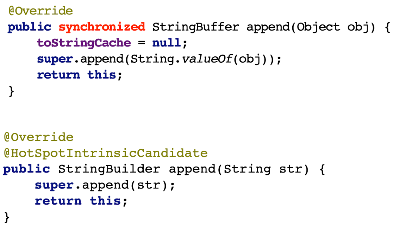
중간에 끼어넣을 수 있는 (문자열 변경이 가능한) 특징을 위한 예제
- buffer.append("bind").append("string");
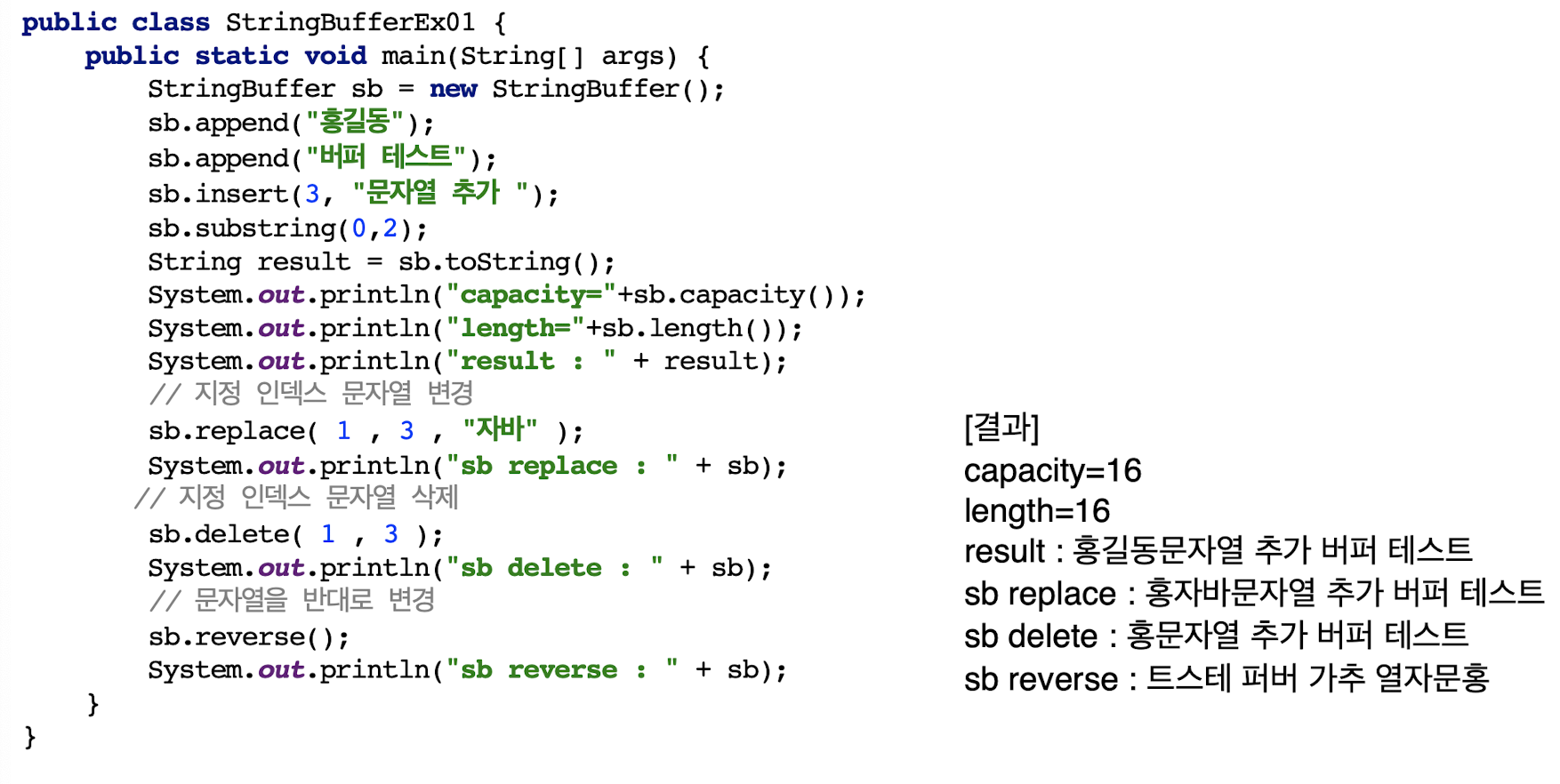
- String vs StringBuffer
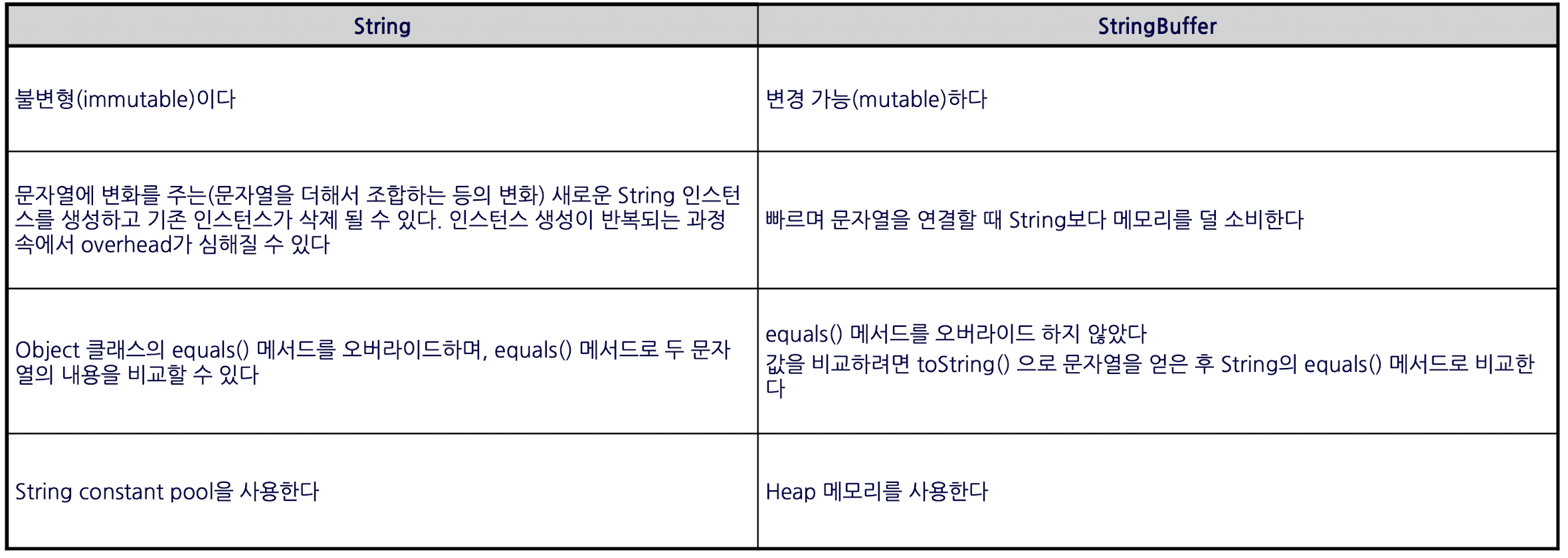
String 클래스에 + 연산하는 경우와 StringBuffer로 +연산하는 경우
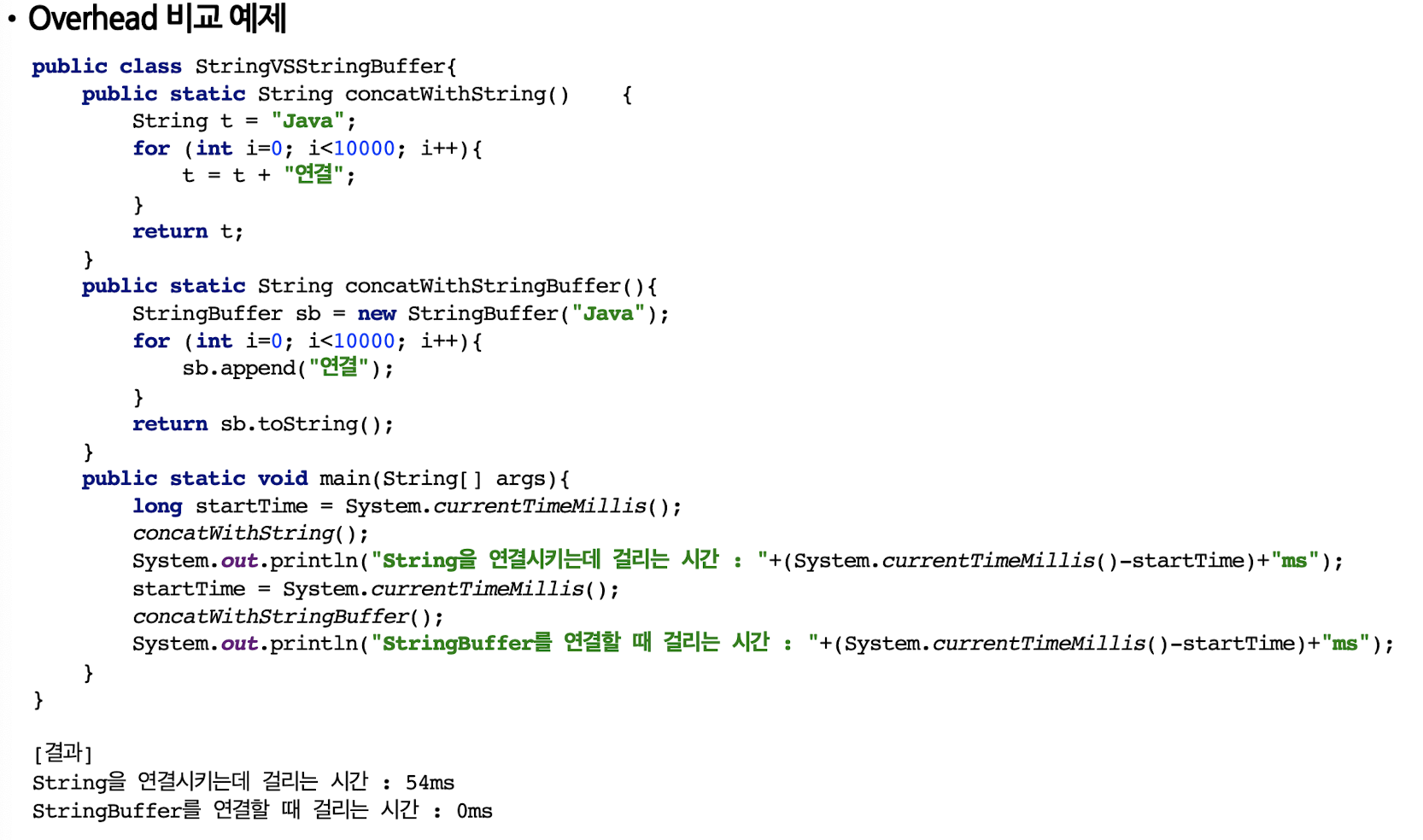
- StringBuffer 메서드
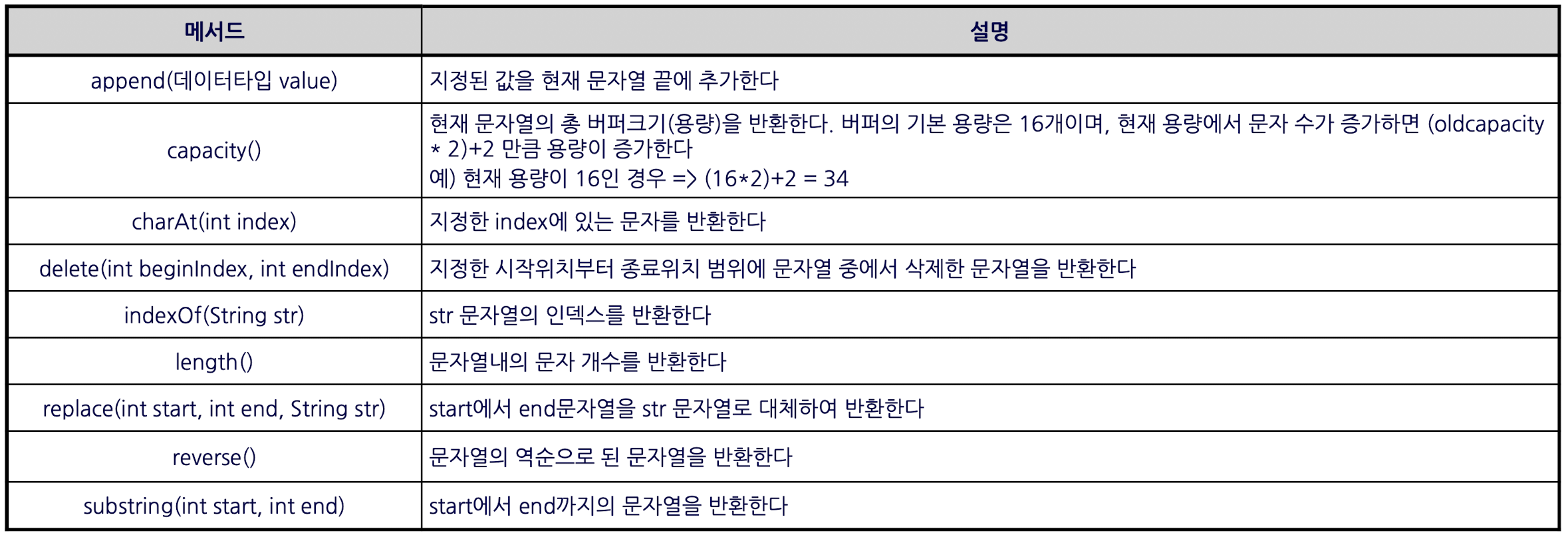
728x90
반응형
'Server Programming > BackEnd Project' 카테고리의 다른 글
35일차 - TIL (0) | 2023.01.16 |
---|---|
32일차 - TIL (0) | 2023.01.13 |
31일차 - TIL (0) | 2023.01.12 |
30일차 - TIL (0) | 2023.01.11 |
30일차 - 자바. 예외처리 (0) | 2023.01.11 |